Salesforce: SMS Connector on Mulesoft Anypoint Studio
This integration guide covers how to set up a connection between the Retarus SMS REST API and Mulesoft Anypoint Studios with Salesforce. For this example, a REST-based service connection is described. However, SOAP and SMTP are also viable options. This approach is also applicable to other Retarus gateways, such as Retarus Cloud Fax and Transactional Email.
Prerequisites
Valid license e.g. Anypoint Platform desktop IDE (Anypoint Studio | Integrated Development Environment (IDE) | MuleSoft)
Valid Retarus REST API Account and Credentials
API Platform, e.g. Postman (Postman API Platform | Sign Up for Free)
Part 1 Logical Setup and Logic Blocks
Logical Setup
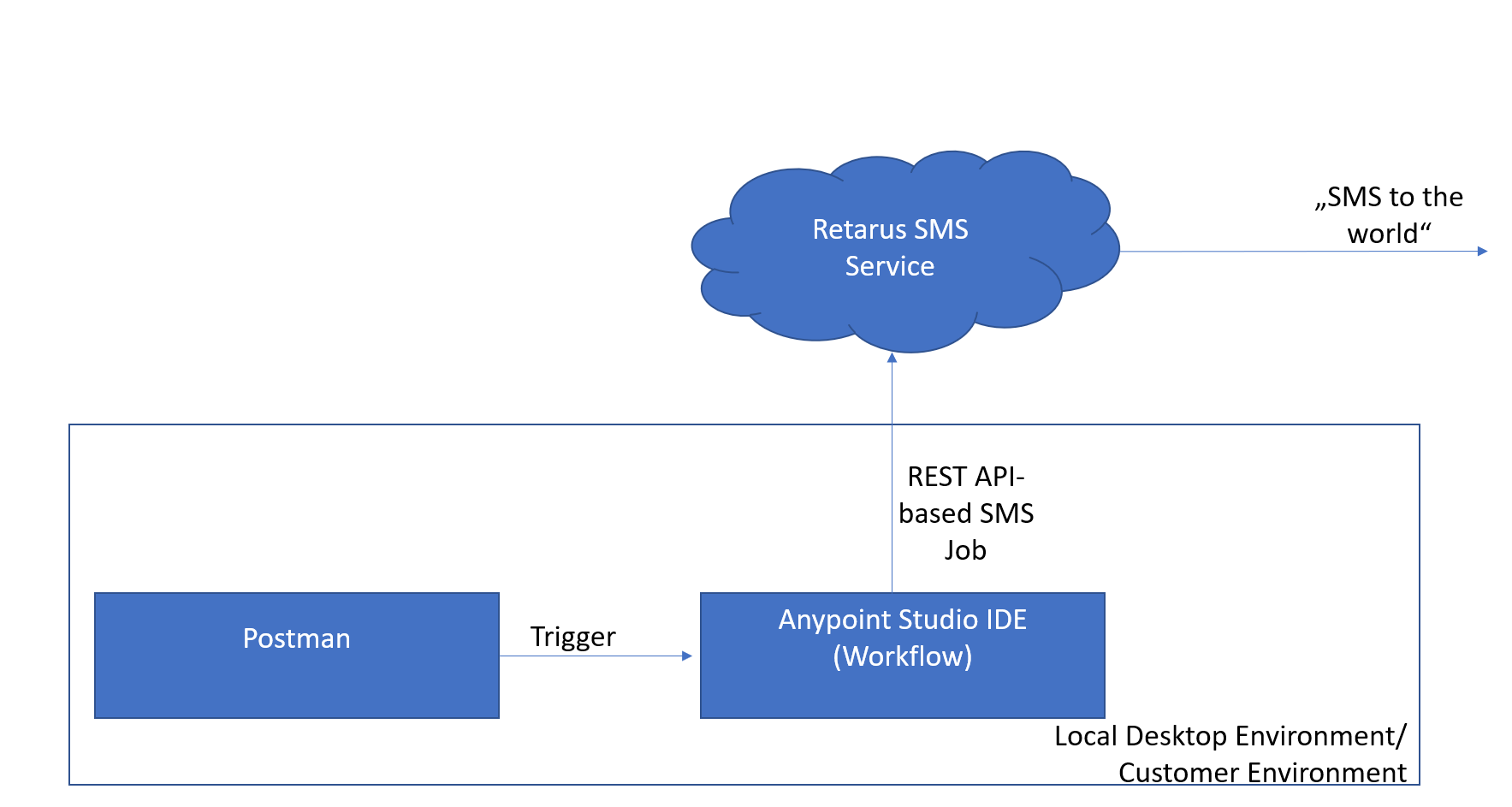
Architectural Overview of the Components
Logic Blocks
Anypoint Studio IDE Logic required blocks:
Anypoint Studio IDE Logic required blocks: listener, transform message, and request
Connecting Salesforce to Mulesoft
Salesforce Flows
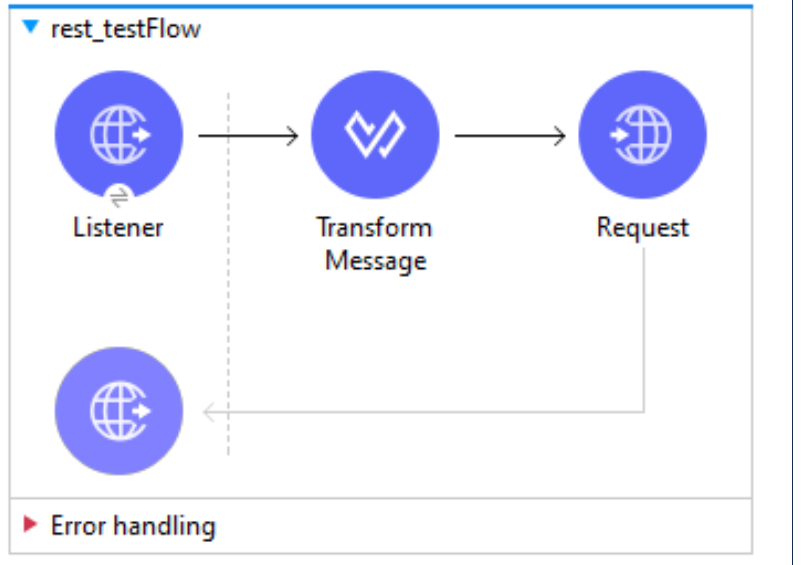
Necessary Logic Blocks
Part 2 Connecting requesting Salesforce application to Mulesoft
Part 3 Send SMS from your Salesforce Application
Programming Logics and Salesforce Flows
The following logics data flows are covered here:
Message parameters are defined by the end-user using the "Dashboard"
The input data is processed by a Salesforce "Flow" Component
The Flow component calls an APEX Class providing via HTTP Request the data to Muleosoft AnyPoint Studio
Mulesoft AnyPoint Studio (compiled program, hosted locally but reachable using NGROX via https request) listens to the HTTP Request von specified Port
AnyPointStudio transforms the received data into the REST API Format required by the Retarus REST API
AnypointStudio sends out an HTTP Request to the Retarus infrastructure