SMS-for-Applications - SOAP
You can automatically send SMS messages using the Retarus SMS-for-Applications Webservice. In addition, you have the option of monitoring the transmission status of your sent messages. The Webservice is accessed via SOAP 1.1 (document/literal) and can only be used via an encrypted SSL HTTP connection.
Webservice URL (WSDL)
https://sms4a.retarus.com/soap/v4/?wsdl
Methods
The Webservice offers the following methods to the client application for sending SMS messages:
Method name | Description |
---|---|
getVersionInfo | Requests the Webservice version. |
sendJob | Sends an SMS job to the Webservice. |
getAvailableJobReports | Requests available SMS job reports. |
getJobReport | Requests a status report for a specific SMS job. |
Authentication & Login IDs
All methods (except getVersionInfo) require authentication with a Login ID and password.
Java sample code
// authenticate
final Request request = factory.createJobRequest();
request.setUsername(your_username);
request.setPassword(your_password);
Upon request, we can configure multiple forms of access (Login IDs) for the Webservice. These Login IDs can be configured and managed via the Retarus EAS Portal. When multiple Login IDs are used, the Webservice can concurrently operate multiple processes and save a configuration for each process.
Method getVersionInfo
The Webservice’s version identifier can be queried using the getVersionInfo method.
Response
Field name | Data type | Required |
---|---|---|
buildNumber | Integer | Yes |
buildTimestamp | String | Yes |
majorVersion | Integer | Yes |
minorVersion | Integer | Yes |
versionInfo | String | Yes |
message | String | No |
buildNumber
Revision number
buildTimestamp
Date created
majorVersion
First component of the version number, e.g., 2016
minorVersion
Second component of the version number, e.g., 6
versionInfo
Additional information
message
Information about this version
Java sample code
// send request
final VersionInfoResponse versionInfo = webservice.getVersionInfo();
// print result
System.out.println(String.format("Build: %s / %05d", versionInfo.getBuildTimestamp(), Integer.valueOf(versionInfo.getBuildNumber())));
Response
Build: 2015-03-26 14:40:52 +0100 / 00054
Method sendJob
This method is used to prepare SMS jobs to be transferred for processing. If a valid JobRequest has been received by the Webservice, the Webservice sends an ID back that must be specified by the client when querying the job status.
Request
Field name | Data type | Required |
---|---|---|
username | String | Yes |
password | String | Yes |
options | Options | Yes |
messages | List <Message> | Yes |
username
Retarus API Login ID
password
Retarus API Password
options
Global job options (see Options below)
messages
SMS messages with different texts and recipients (see Message).
Requests which result in more than 3.000 SMS (based on several messages or recipients) will be rejected.
Options
Field name | Data type | Required |
---|---|---|
src | String | No (Default value EAS*) |
encoding | String | No (Default value EAS*) |
billcode | String | No (Default value EAS*) |
statusRequested | Boolean | No (Default value EAS*) |
flash | Boolean | No (Default value EAS*) |
customerRef | String | No (Default value EAS*) |
validityMin | Long | No (Default value EAS*) |
maxParts | Integer | No (Default value EAS*) |
qos | String | No (Default value EAS*) |
jobPeriod | String | No (Default value immediately) |
duplicateDetection | Boolean | No (Default value false) |
blackoutPeriods | List <String> | No (Default value none) |
(*) signifies that the option has not been added to the job, which means the default value configured in EAS will be applied.
src
The Sender ID displayed to recipient. When entering the Sender ID, please note the following technical restrictions:
If the Sender ID is exclusively numerical, a maximum of 20 numbers can be used. A + character is available for optional use.
If alphanumeric characters are used, the maximum length is 11.
The maximum ranges of characters that can be selected between the first and last letters are:
TEXTa-z, A-Z, 0-9, !"#$%&'()*+,-./:;=<>?@[\]^_`{|}~
No other characters are permitted. Java Regular Expressions:
TEXT(.\\+|)[0-9]{1,20}|[a-zA-Z0-9\\x20\\p{Punct}]{1,11}
p{Punct}
stands for the following: !"#$%&'()*+,-./:;<=>?@[\]^_`\{|}~
Invalid Sender IDs are corrected automatically (e.g., replaced with an X)
encoding
The preferred encoding of an SMS. Potential values: GSM-7 (standard) or UTF-16 (alternate). When GSM-7 is selected, the Webservice checks SMS messages for invalid characters. You can configure how invalid characters will be handled in the EAS portal (see Configuring SMS-for-Applications).
The selection of encoding impacts the maximum length of the SMS.
Important information regarding SMS coding using GSM-7:
There is a maximum of 160 characters per single-part SMS
A maximum of 153 characters per SMS in a multi-part SMS
Some special characters, such as, e.g., €, require additional storage capacity (2 normal characters)
Important information regarding SMS encoding with UTF-16:
A maximum of 70 characters when sending a single-part SMS
A maximum of 67 characters per SMS in a multi-part SMS
In some countries, an SMS will not reach the recipient if it contains special characters.
Please consult your sales engineer for additional information.
billcode
Optional billing information can be entered here.
Max. 70 characters
statusRequested
Requests a delivery notification.
An additional fee is required for this option.
flash
You can set here whether you want the SMS sent as a Flash SMS, which is sent directly to the recipient’s mobile phone display without the recipient explicitly having to open it.
An additional fee is required. The Flash SMS option is not supported in all countries.
customerRef
Optional reference information that will be included in getJobReport.
Max. 70 characters (192 for US-ASCII encoding).
validityMin
Expresses the validity of an SMS in minutes and thus the length or the delivery attempt when an SMS cannot instantly be delivered after being sent (e.g., if the recipient’s mobile phone is shut off). Supported values are between 5 and 2880 minutes. If the specified value is outside of the range of permitted values, either the minimum or maximum value will automatically be selected. Exception: 0, in which case the provider’s default value is used.
maxParts
Sets the maximum amount of SMS in a multi-part (concatenated) SMS. If the SMS message is longer, it is truncated. Permitted values: from 1 to 20. If the specified value is outside of the range of permitted values, either the minimum or maximum value will automatically be selected. The actual length of an SMS is dependent on the selected encoding standard (see encoding earlier in this section for additional information).
Multi-part SMS are not supported in CDMA networks.
qos
Sets the priority of an SMS job. If you have time-critical SMS messages, we recommend that you select the express option. Supported values are: normal and express.
An additional fee is required to send an express SMS.
jobPeriod
These periods are based on the ISO-standard 8601; e.g., Z can be +02:00.
If the jobPeriod syntax is invalid, the job is rejected.
Examples:
TEXT2016-08-01T13:00:00Z 2016-08-01T13:00:00+02:00
duplicateDetection
If enabled, equal requests are rejected by throwing a DuplicateJobException.
The equality is determined from the SOAP request data.
Currently the time window for duplicate detection is 10 minutes. This means that after 10 minutes the same job can be successfully sent once again.
This parameter should normally be enabled if the customer’s system erroneously generates duplicate requests.
blackoutPeriods
Specifies time periods during which no SMS are delivered; e.g., if a delivery time is inside such a period, the SMS is scheduled to be sent at the end of the period.
These periods are based on the ISO 8601 standard.
Periods that are smaller than 1 hour are expanded to 1 hour (e.g.,: 17:10 - 17:20 is expanded to 17:10 - 18:10)
If the blackoutPeriods syntax is invalid, the job is rejected. Examples:
2015-03-25T18:00Z/2015-03-26T07:00Z
2015-03-25T18:00+01:00/2015-03-26T07:00+01:00
Message
Field name | Data type | Required |
---|---|---|
text | String | Yes |
recipients | List <Recipient> | Yes |
text
The SMS message
recipients
Recipient List must have a minimum quantity of 1 (see Recipient)
Recipient
Field name | Data type | Required |
---|---|---|
dst | String | Yes |
customerRef | String | No |
blackoutPeriods | List <String> | No |
dst
Recipient’s mobile phone number. If a number is specified without a country code, the value specified in the EAS configuration parameter Country code will automatically be applied.
customerRef
Discretionary reference information. If no value is specified, the recipient mobile phone number specified in the dst field will be applied. This information is included in the status report.
Max. 70 characters.
blackoutPeriods
Specifies time periods during which no SMS are delivered. E.g., if a delivery-time is inside of such a period, it is rescheduled to the end of the period.
Response
Field name | Data type | Required |
---|---|---|
jobId | String | Yes |
Java sample code (minimum)
// authenticate
final JobRequest request = factory.createJobRequest();
request.setUsername(your_username);
request.setPassword(your_password);
// message + recipients
final Message message = factory.createMessage();
message.setText("Hello Sms4A-SOAP-Service");
final Recipient recipient = factory.createRecipient();
recipient.setDst("+4917600000000");
message.getRecipients().add(recipient);
// add message to request
request.getMessages().add(message);
// send to retarus sms cloud infrastructure
final JobResponse response = webservice.sendJob(request);
// print result
System.out.println(response.getJobId());
Java sample code (maximum)
// authenticate
final JobRequest request = factory.createJobRequest();
request.setUsername(your_username);
request.setPassword(your_password);
// options
final Options options = factory.createOptions();
options.setSrc("TheSender");
options.setEncoding("utf-16");
options.setBillcode("BillingCode");
options.setStatusRequested(Boolean.TRUE);
options.setFlash(Boolean.FALSE);
options.setCustomerRef("JREF.1234567890");
options.setValidityMin(Long.valueOf(10));
options.setMaxParts(Integer.valueOf(2));
options.setQos("normal");
options.setJobPeriod("2014-09-18T12:09:58.246Z");
options.setDuplicateDetection(Boolean.TRUE);
options.getBlackoutPeriods().add("2015-03-25T18:00+01:00");
request.setOptions(options);
// message 1 + recipients
final Message message1 = factory.createMessage();
{
message1.setText("Hello Sms4A-SOAP-Service");
final Recipient recipient1 = factory.createRecipient();
recipient1.setDst("+491760100000000");
recipient1.setCustomerRef("RREF.00001");
recipient1.getBlackoutPeriods().add("2015-03-26T07:00+01:00/2015-03-26T08:00+01:00");
recipient1.getBlackoutPeriods().add("2015-03-26T12:00+01:00/2015-03-26T14:00+01:00");
final Recipient recipient2 = factory.createRecipient();
recipient2.setDst("+491760200000000");
recipient2.setCustomerRef("RREF.00002");
message1.getRecipients().add(recipient1);
message1.getRecipients().add(recipient2);
}
// message 2 + recipients
final Message message2 = factory.createMessage();
{
message2.setText("Hello there...");
final Recipient recipient3 = factory.createRecipient();
recipient3.setDst("+491760300000000");
recipient3.setCustomerRef("RREF.00003");
message2.getRecipients().add(recipient3);
}
// add messages to request
request.getMessages().add(message1);
request.getMessages().add(message2);
// send to retarus sms cloud infrastructure
final JobResponse response = webservice.sendJob(request);
// print result
System.out.println(response.getJobId());
Response
J.20130507-141110.189-0hgf1et4eDX9IAVNV1
Method getAvailableJobReports
This method delivers a list of Job IDs from a specific time period. Job IDs up to 3 months old can be retrieved.
Request
Field name | Data type | Required |
---|---|---|
username | String | Yes |
password | String | Yes |
fromTs | Date | Default: going back 14 days from today |
toTs | Date | Default: now |
offset | Integer | Default: 0 |
limit | Integer | Default: 100 |
open | Boolean | Default: nothing |
username
Retarus API Login ID.
password
Retarus API Password.
fromTs
From timestamp (maximum 30 days before toTs).
toTs
To timestamp (must be after fromTs).
offset
If the number of results is larger than the limit set for it, with the assistance of the offset you can query more recent results, or skip over a specified number of Job IDs.
limit
Limits the results list to a specific number of Job IDs (0 < limit ⇐1000).
open
Restricts the results list to Job IDs that are either still open or have already been completed (blank = both conditions).
Response
Field name | Data type | Required |
---|---|---|
jobIds | List <String> | Yes |
Java sample code (minimum)
// authenticate
final AvailableJobReportsRequest request = factory.createAvailableJobReportsRequest();
request.setUsername(your_username);
request.setPassword(your_password);
// send request
final AvailableJobReportsResponse availableJobReports = webservice.getAvailableJobReports(request);
// print result
for (final String id : availableJobReports.getJobIds()) {
log.info(id);
}
Java sample code (maximum)
// authenticate
final AvailableJobReportsRequest request = factory.createAvailableJobReportsRequest();
request.setUsername(your_username);
request.setPassword(your_password);
// parameter
request.setFromTs(dataTypeFactory.newXMLGregorianCalendar(2013, 06, 01, 10, 0, 0, 0, timeZone));
request.setToTs(dataTypeFactory.newXMLGregorianCalendar(2013, 06, 01, 11, 0, 0, 0, timeZone));
request.setOffset(Integer.valueOf(0));
request.setLimit(Integer.valueOf(100));
request.setOpen(Boolean.TRUE);
// send request
final AvailableJobReportsResponse availableJobReports = webservice.getAvailableJobReports(request);
// print result
for (final String id : availableJobReports.getJobIds()) {
log.info(id);
}
Response
Job IDs in ascending order.
...
J.20130507-114341.980-0hgew55stNJ43O23CO
J.20130507-114353.692-0hgew5eu5DU8L30KMD
J.20130507-115418.142-0hgewisnzVWALCVZIZ
J.20130507-130914.189-0hgez75u6L3X9LKXB3
J.20130507-181443.092-0hgfa40idP4Z2Y7IUH
J.20130507-181619.939-0hgfa638k85IODDXGG
...
Method getJobReport
With the assistance of this method, an SMS job’s status report can be requested by Job ID. Status reports can be retrieved for up to 3 months.
Request
Field name | Data type | Required |
---|---|---|
username | String | Yes |
password | String | Yes |
jobId | String | Yes |
username
Retarus API Login ID.
password
Retarus API Password.
jobId
Job ID.
Response
The response will contain the fields described in the table below, with the default content type "application/xml" and charset "UTF-8". Please ensure that your application can process the data in this format. If you want to receive responses with content type "application/json", please contact your Retarus Account Manager or the Retarus Service Desk.
Field name | Data type | Required |
---|---|---|
jobId | String | Yes |
src | String | Yes |
encoding | String | Yes |
billcode | String | Yes |
statusRequested | Boolean | Yes |
flash | Boolean | Yes |
validityMin | Long | Yes |
customerRef | String | Yes |
qos | String | Yes |
receiptTs | Date | Yes |
finishedTs | Date | No |
recipients | List <RecipientWithStatus> | Yes |
jobId
Job ID.
src
Sender ID.
encoding
Text encoding.
billcode
Billing code.
statusRequested
Delivery notification requested.
flash
Send as an important SMS that automatically appears on the recipient’s display screen.
validityMin
Validity of the SMS in minutes.
customerRef
Customer reference text (default is null).
qos
Quality of Service.
receiptTs
Date the job was received.
finishedTs
Date the job was completed.
recipients
List of the recipients of the job.
RecipientWithStatus
Field name | Data type | Required |
---|---|---|
dst | String | Yes |
customerRef | String | Default: original <dst> |
smsId | String | Yes |
processStatus | String | Yes (see Status descriptions for further information). |
status | String | Yes (see Status descriptions for further information). |
reason | String | Default: <null>; see Status descriptions for further information. |
numberofParts | Integer | Yes |
sentTs | Date | Default: <null> |
finishedTs | Date | Default: <null> |
dst
Recipient’s mobile phone number. If a number is specified without a country code, the value specified in the EAS configuration parameter Country code will automatically be applied.
customerRef
Discretionary reference information. If no value is specified, the recipient mobile phone number specified in the dst field will be applied. This information is included in the status report. Please note: Max. 64 characters.
SmsId
The unique ID for the SMS.
processStatus
See Status descriptions.
status
See Status descriptions.
reason
See Status descriptions.
numberofParts
The number of SMS parts the message consists of.
sentTS
Timestamp which indicates when the SMS was sent.
finishedTS
Timestamp which indicates when the SMS received its final status.
Status descriptions
ProcessStatus | Status | Description | statusRequested |
---|---|---|---|
OPEN | <empty> | Pending SMS, not yet scheduled. | <Irrelevant> |
OPEN | <any> | In re-try state (e.g., during a connection error to service provider). | <Irrelevant> |
DONE | SUC_ACC | Successful ( without delivery notification). | False |
DONE | SUC_FIN | Successful (with delivery notification). | True |
DONE | INVALID_DST | Invalid number (e.g., 999999). | <Irrelevant> |
DONE | BLOCKED | Blocklisted number. | <Irrelevant> |
DONE | TIMEOUT | Sent, but the validity period was exceeded. | True |
DONE | CON_ERR | Failed; all retry attempts were unsuccessful. | <Irrelevant> |
DONE | FAILED_FIN | Failed; delivery notification returns this status. | True |
DONE | NOT_ACC_FIN | Failed; delivery to the provider was/is not possible. | <Irrelevant> |
DONE | SEND_ERR | Internal error (in most cases, the SMS has arrived anyway). | <Irrelevant> |
DONE | STATUS_ERR | Internal error during the query for the delivery notification. | True |
CANCELED | <empty> | Transmisson was cancelled before the initial delivery attempt. | <Irrelevant> |
CANCELED | NOT_ACC_REC | Transmisson was cancelled after the initial delivery attempt. | <Irrelevant> |
RUNNING | <any> | SMS was sent, but no delivery notification was received. | True |
KILL | <any> | SMS was sent, but cancelled and no delivery notification has been received. | True |
Java sample code
// authenticate
final JobReportRequest request = factory.createJobReportRequest();
request.setUsername(your_username);
request.setPassword(your_password);
// jobid
request.setJobId("J.20130514-162552.561-0hgp6b06qGV0I35D5T");
// send request
final JobReportResponse report = webservice.getJobReport(request);
for (final RecipientWithStatus recipient : report.getRecipients()) {
System.out.println(String.format("%s / %s / %s", recipient.getDst(),
recipient.getProcessStatus(), recipient.getStatus()));
}
Response
...
+4910001100000000 / OPEN / <null>
+4910002100000000 / DONE / SUC_FIN
+4910003100000000 / DONE / SUC_FIN
+4910004100000000 / DONE / SUC_FIN
+4910005100000000 / DONE / TIMEOUT
...
Status callback
You can configure automatic push of report data to your application in the EAS portal.
If your application is temporarily not reachable, there will be three retries in the first minute, an additional nine retries in the first 10 minutes, and a further retry every 15 minutes for approximately 48 hours. You also have the option of obtaining the data using the methods described in Method getJobReport (SOAP) and Getting information about all recipients of a job (REST).
Please ensure that your application can process the data as specified in Method getJobReport (SOAP) and JobReport (REST). The response will be identical to that in the JobReportResponse.
EAS configuration
You can change your API access password and configure default values in the EAS portal. Default values (displayed in parentheses in Figure 1) are applied if the corresponding option is not defined within a job. The following section explains how to configure SMS-for-Applications in EAS. Additional information on the EAS portal is available in Classic EAS Portal.
Configuring SMS-for-Applications
Begin by logging in to the EAS portal. In the EAS navigation menu on the left-hand side of the screen, click sequentially on Administration - SMS Services - SMS for Applications and then select your Login ID (it will already have been created for you). All changes are saved immediately.
It may take a few minutes for changes to take effect.
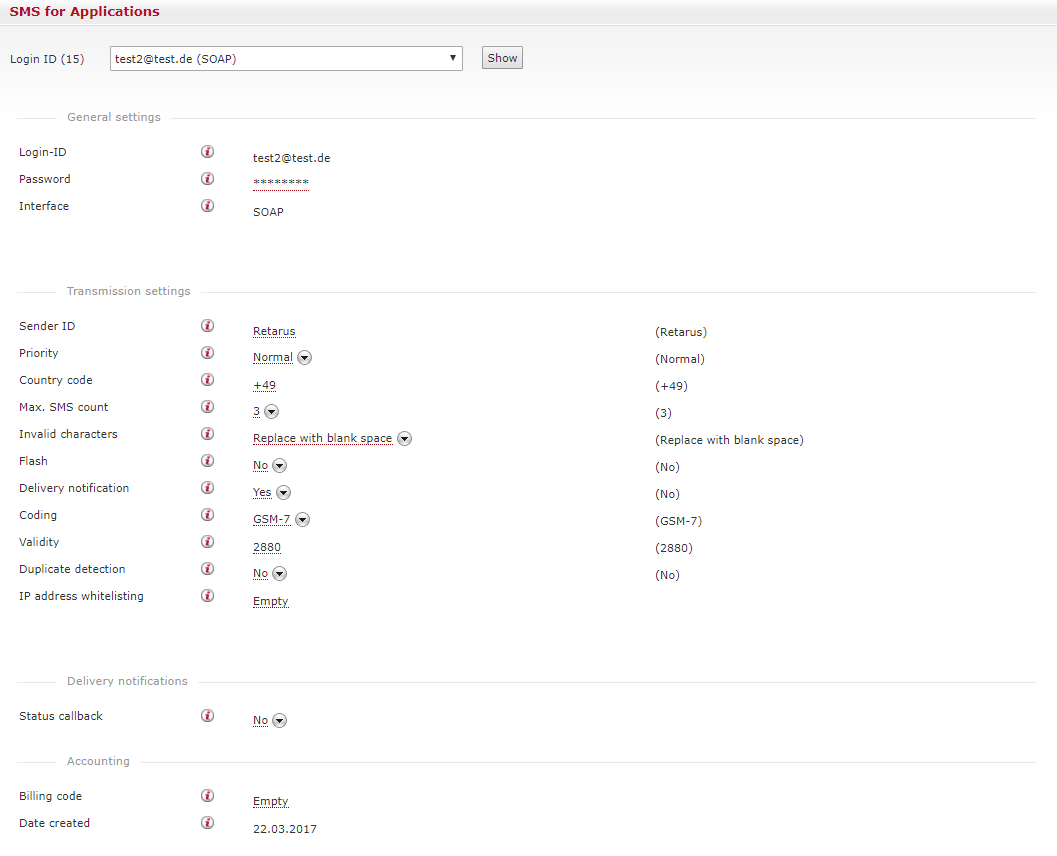
General settings
The following table explains the functions of the fields in the General settings section:
Field name | Description |
---|---|
Login ID | Not alterable. |
Password | Must be at least 8 characters long and contain upper- and lower-case letters as well as numerical digits and special characters (e.g., !MyPassword12). |
Interface | Depends on which service has been booked (REST, SOAP, or XML). |
Transmission settings
The following table explains the functions of the fields in the Transmission settings section:
Field name | Description |
---|---|
Sender ID | Displayed to the recipient. If alphanumeric, it is limited to 11 characters. |
Priority | Can be either Normal or Express. Default is Normal. The use of the Express option requires an additional fee. |
Country code | If the SMS job is sent to the Webservice without a country code, the one in this field is added as a prefix. The local country code is the default setting. |
Max. SMS count | Limits the number of separate SMS in a multi-part SMS. Max value is 20. |
Invalid characters | If any characters in an SMS message cannot be displayed with the selected encoding, there are four options for dealing with them: Deny (meaning that the SMS job is not executed), Replace with blank space (the default), Convert to UTF-16, and Smart Replacement (in which invalid characters are replaced by the next characters in the GSM-7 character set). When Convert to UTF-16 is selected under Invalid characters, the maximum SMS length is shortened (it was longer with GSM-7) and messages may get truncated. If the Max. SMS count field is greater than 1, it is possible that more SMS will be sent than with GSM-7 encoding, resulting in additional costs. |
Flash | Sends a message as a Flash SMS. Flash SMS is not available in all countries and depends on the carrier and end device. Default is No. The use of this option requires an additional fee. |
Delivery notification | Requests a delivery notification. Default is No. The use of this option requires an additional fee. |
Coding | GSM-7 or UTF-16. With GSM-7, there is a limit of 160 characters per single-part SMS and 153 per part in a multi-part SMS. With UTF-16, there is a limit of 70 characters per single-part SMS and 67 per part in a multi-part SMS. |
Validity | Validity in minutes of an SMS message. Potential values are between 5 and 2880. |
Duplicate detection | This feature prevents recipients from receiving an identical message within a ten minute periode. |
IP address whitelisting | Comma separated list of IP addresses or subnets which should be allowed to access the service. If this is configured, all other IP will be blocked. Maximum 50 Items are allowed. Example |
Delivery notifications
The following table describes the configuration settings for the Delivery notifications features:
Field name | Description |
---|---|
Status callback | Select Yes to activate the push of delivery notification to the application. No deactivates the push. |
Callback URL | The URL of the application to which the delivery notification status is pushed. |
Authentication method | Select an authentication method from the dropdown menu: options are None, Basic, or Digest. |
User | Enter the name of the user for authentication purposes. |
Password | Has to contain 8 characters, including capital- and lower-case letters, as well as numbers and special characters. |
HTTP method | Select either POST or PUT from the dropdown menu. |
Accounting settings
The following table explains the functions of the fields in the Accounting section:
Field name | Description |
---|---|
Billing code | You can opt to enter billing information here. |
Date created | Date the profile was created. |
SMS push services
In addition to transmission of SMS messages, Retarus SMS-for-Applications also offers the option of pushing inbound SMS messages directly to applications, which enables two-way communication with customers and/or employees.
More details and individual configuration options for existing services can be found in Inbound Settings.